Getting started
The GPXZ API provides worldwide elevation data via a REST API.
This page is a quickstart guide to accessing elevation data through the GPXZ API. After that, you might want to learn more about how our dataset is made, or check out the API reference for the different ways to access elevation data.
Authentication
An API key is needed to access the GPXZ API. After signing up for a free account, you can copy your key API keys tab in the dashboard. It will be a bunch of random characters prefixed by ak_
, like in this screenshot:
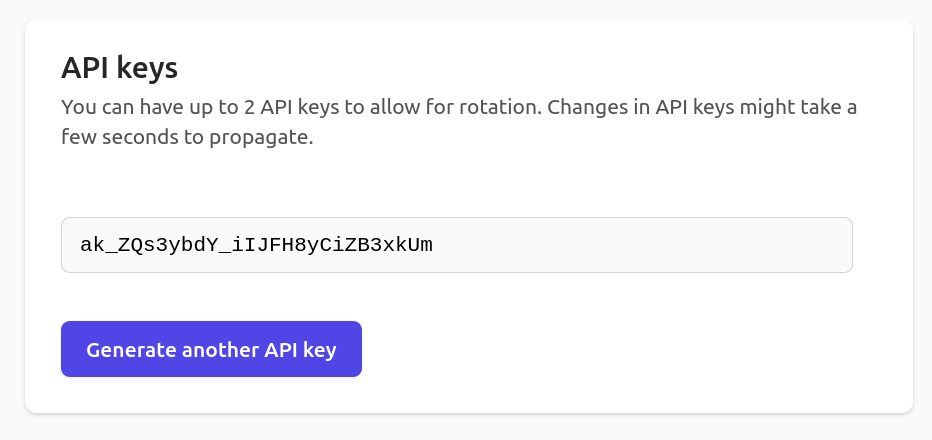
You can pass the key in the api-key
query parameter when building a GPXZ url. For example, to get the elevation of Uluru at (-25.345, 131.034) you would use the url:
https://api.gpxz.io/v1/elevation/point?lat=-25.345&lon=131.034&api-key=YOUR-API-KEY-HERE
URL parameters are great for quick browser testing! But in production it's more secure to pass the key as the x-api-key
header: unlike URLs, headers aren't typically logged. In Python, this might look like
import requests
API_KEY = "YOUR-API-KEY-HERE"
response = requests.get(
"https://api.gpxz.io/v1/elevation/point?lat=-25.345&lon=131.034",
headers={"x-api-key": API_KEY},
)
print(response.json())
Rate limiting
To ensure stability and performance for all users, GPXZ imposes some limits on how fast you can make requests. There's a requests-per-second limit, and a requests-per-day limit. You can see your account's limits on your dashboard:

The requests-per-second limit is enforced for all users. Requests above the limit receive a response with a 429 status code: these requests don't count towards your quota, so feel free to retry rate-limited requests until they succeed.
For free plans, the requests-per-day limit is enforced. It resets midnight UTC. You can find your current daily request total on your dashboard under "Recent Usage".
For customers with paid accounts, the requests-per-day limit is not enforced: we understand that usage varies day to day and never want to cut you off. If you're regularly exceeding the posted limit, we'll reach out and talk about upgrading to a larger plan.
Seeing your realtime usage
To help you understand your realtime usage, the GPXZ API adds these headers to most responses:
x-ratelimit-used
: number of API requests made todayx-ratelimit-limit
: your requests-per-day quotax-ratelimit-remaining
: requests you can make until exceeding the requests-per-day quotax-ratelimit-reset
: seconds until the daily request limit resetsretry-after
(only for 429-status responses): seconds until the per-day or per-second limit resets, whichever triggered the 429 response.
These headers may not all be present on non-200 response status requests (like client errors), and on unauthenticated endpoints (like GET /v1/health).
EU region
By default, API requests are processed on a cluster of servers located in different regions around the world.
If instead you would like your requests to be handled only by servers within the EU, you can replace our standard domain api.gpxz.io
with api-eu.gpxz.io
- Accounts can use both the EU and Global regions simultaneously.
- Requests made to the EU region from clients outside the EU may go through non-EU servers from our load balancer Cloudflare. You can read more about Cloudflare's EU privacy stance and our privacy policy.
- Irrespective of the subdomain used, customer PPI (e.g., user details, logs, metrics) are only stored and processed in the EU.
Errors
GPXZ error responses look like this:
{
"status": "INVALID_REQUEST",
"error": "This endpoint doesn't exist."
}
There is a status
property with a value either of INVALID_REQUEST
for 4xx response codes, and SERVER_ERROR
for 500 response codes (a successful response will have a status
of OK
).
And there is an error
property with a plain-language description of what went wrong.
Server errors (500 responses) are rare and will typically succeed if retried. We get notified of and fix every 500 response!
Rate-limited 429 errors may be resolved by retrying with backoff.
All other client (4xx) errors are unlikely to be resolved by retrying, you'll need to fix the request.
Units and coordinate systems
- Longitudes should be in the range [-180, 180].
- All latitude/longitude coordinates (inputs and outputs) are in EPSG:4326 (aka WGS84) format.
- The GPXZ dataset doesn't have any holes or missing data, so the API will never return
null
(orInfinity
orNaN
) as an elevation. - Elevations values are returned with lots of decimal points. This is for implementation simplicity and interpolation smoothness, not because the data is millimetre-accurate! You should round numbers before displaying to end users.
- The GPXZ dataset is regularly updated. The version of the dataset used is returned with all requests in the `X-DATASET-VERSION` response header.